Learning Goals
At the end of this Tutorial, you will be able to:
- Run the create-react-app utility and be familiar with its folder and file structure.
- Customise the template files according to your requirements.
- Build a basic app with functional components, and imported CSS and image files.
About Create React App (CRA)
One way to build a Single Page Application (SPA) with React is to use the official command-line utility called create-react-app.
It sets up your development environment so that you can use the latest JavaScript features, provides a nice developer experience, and optimises your app for production.
Building your first app with CRA
Follow these steps to work with create-react-app.
- In a Command Prompt window or inside a VS Code Terminal, navigate to where you want ReactJS to create a folder for your app. For example:
C:\> users\JohnSmith
orC:\>
- Type the following command that includes the name you want to call your new app.
npx create-react-app hello-react
Over a few minutes, React will respond as shown below.
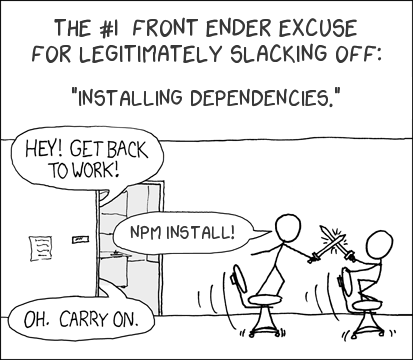
When the process is complete, you will see the following message.
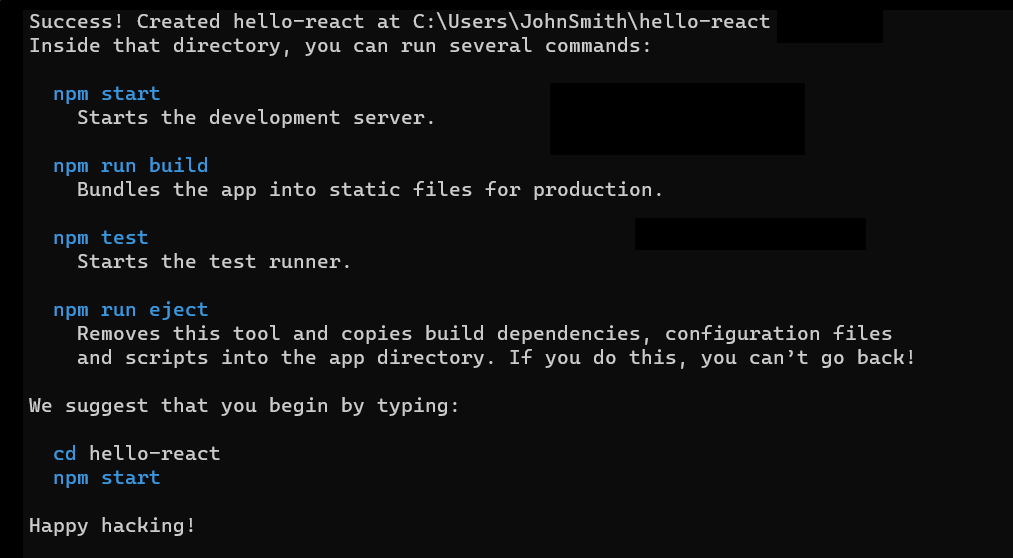
Starting your ReactJS app
After you have created your app, you launch it by running the following command.
npm start
You must run this command from inside your new app folder. For example:
C:/Users/JohnSmith/hello-react> npm start
You will see a number of messages that end as shown below.
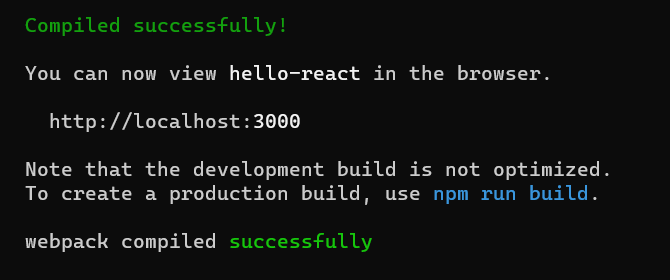
A new browser window should display with your new app running on the React local development server. You will see a screen similar to the following.
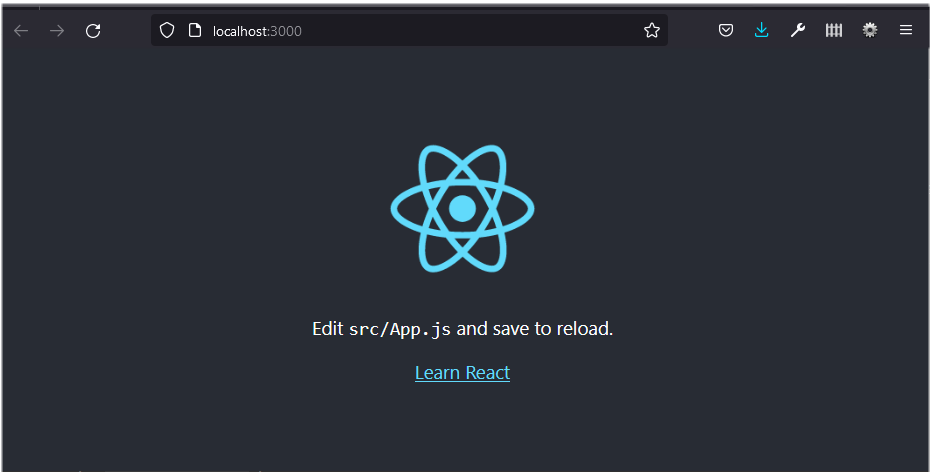
If a new browser tab does not open automatically, open a new tab and enter http://localhost:3000.
ReactJS folders and files
React will create the following hierarchy of folders and files.
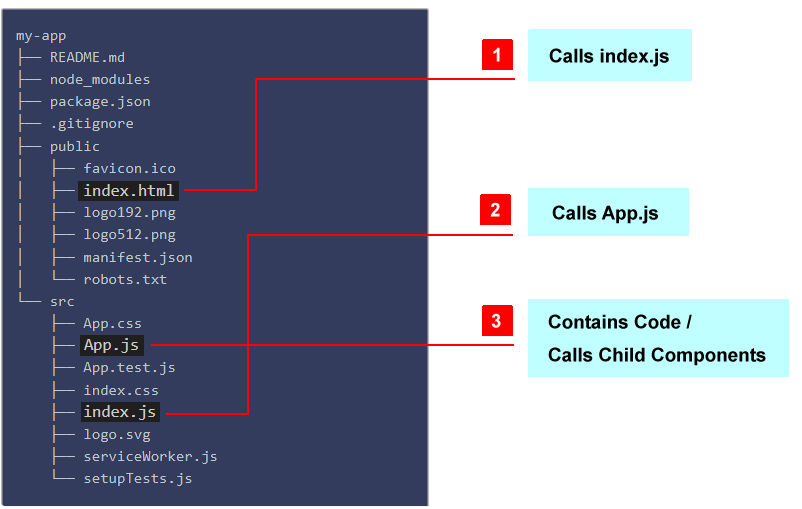
The items you need to know about for creating a simple app are as listed below.
The /public
folder
Your app's root folder that gets served up to the user.
index.html |
The web page that is served when your app is run. It has a <div id="root"></div> container into which index.js injects the top-level or 'parent' <App /> component. |
The /src
folder
This contains your app's components, CSS files, images, and so on. All of the code you write will be located here.
index.js |
The entry point for your app. It contains the ReactDOM.render() statement that injects the top-level <App /> component into the root container of index.html. |
App.js |
This contains the top-level <App /> component of your app. |
Your App.js file may contain all the code for your ReactJS app. But more commonly, it acts as a 'parent' component that imports other, single-purpose 'child' components.
Customising your app
Next, you will customise the 'boilerplate' content provided by the create-react-app utility to build a very basic React app.
- Open the index.html file in the /public folder and replace all its content with the following.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <title>Minimal React App</title> <meta name="description" content="Minimal website created using create-react-app" /> <link rel="manifest" href="%PUBLIC_URL%/manifest.json" /> </head> <body> <div id="root"></div> </body> </html>
- Open the index.js file in the /src folder and replace all its content with the following.
import React from 'react'; import ReactDOM from 'react-dom/client'; import App from './App'; const root = ReactDOM.createRoot(document.getElementById('root')); root.render( <React.StrictMode> <App /> </React.StrictMode> );
- Open the App.js file in the /src folder and replace its content with the following.
function App() { return ( <> <h1>Hello, World!</h1> <p>Paragraph of text.</p> </> ); } export default App;
Your web page should now look as shown below.

In this basic app, you have added code directly to the App.js file.
Adding child components
Next, you will add three components to your app. When finished, your App.js file will be the 'parent' component and three others will all be 'child' components.
- In the /src folder, create a Header.js file with the following content.
function Header() { return ( <header><h1>Corporate strategy</h1></header> ) } export default Header;
- Also in the /src folder, create a Main.js file with the following content.
function Main() { return ( <main><section><p>We leverage agile frameworks to provide a robust synopsis for high-level overviews. Iterative approaches to corporate strategy foster collaborative thinking to further the overall value proposition.</p></section></main> ) } export default Main;
- And finally in the /src folder, create a Footer.js file with the following content.
function Footer() { return ( <footer><p>ABC Corporation. Digital Consultants</p></footer> ) } export default Footer;
- Now, update your App.js file so that it acts as the 'parent' to your three new components. See below.
import Header from './Header'; import Main from './Main'; import Footer from './Footer'; function App() { return ( <> <Header /> <Main /> <Footer /> </> ); } export default App;
Your web page should now look similar to the following.
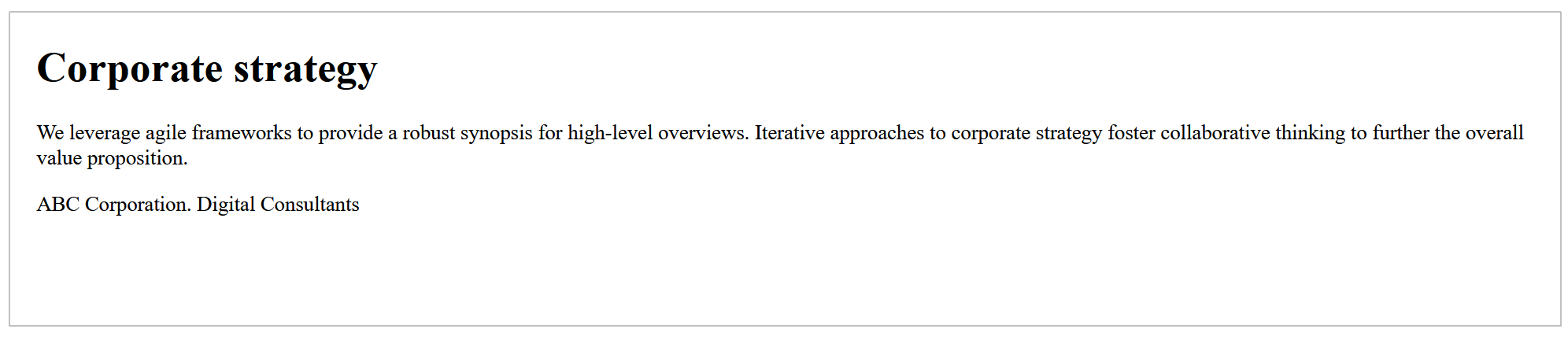
The parent-child relationships between the various files in your ReactJS app are illustrated below.
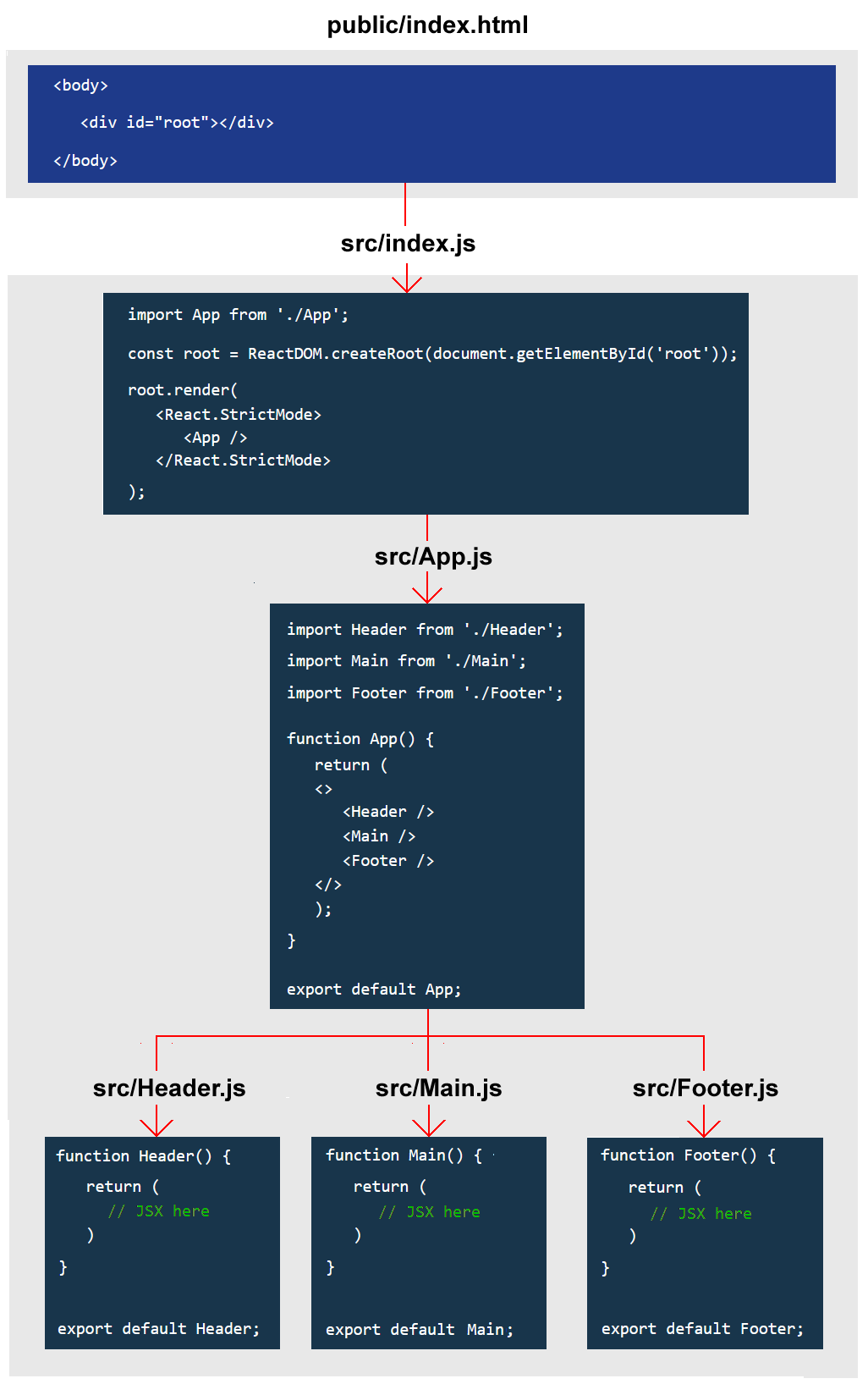
Adding a CSS file
In non-React websites, you would typically add a CSS file by including a link to it in the <head>. For example.

For a React app, you could include a similar link in the index.html file in the /public folder.
However, this folder is ignored by the WebPack bundler when outputting a production-ready version of your app. For this reason, it is better to place your CSS file(s) and any images in your /src folder (or a sub-folder of it) and import them with JavaScript.
See the steps below.
- Download the following file to your /src folder. Minimal.css
- Add a new import statement to the three already in your App.js file as follows.
import Header from './Header'; import Main from './Main'; import Footer from './Footer'; import './Minimal.css';
Your web page should now look as shown below.
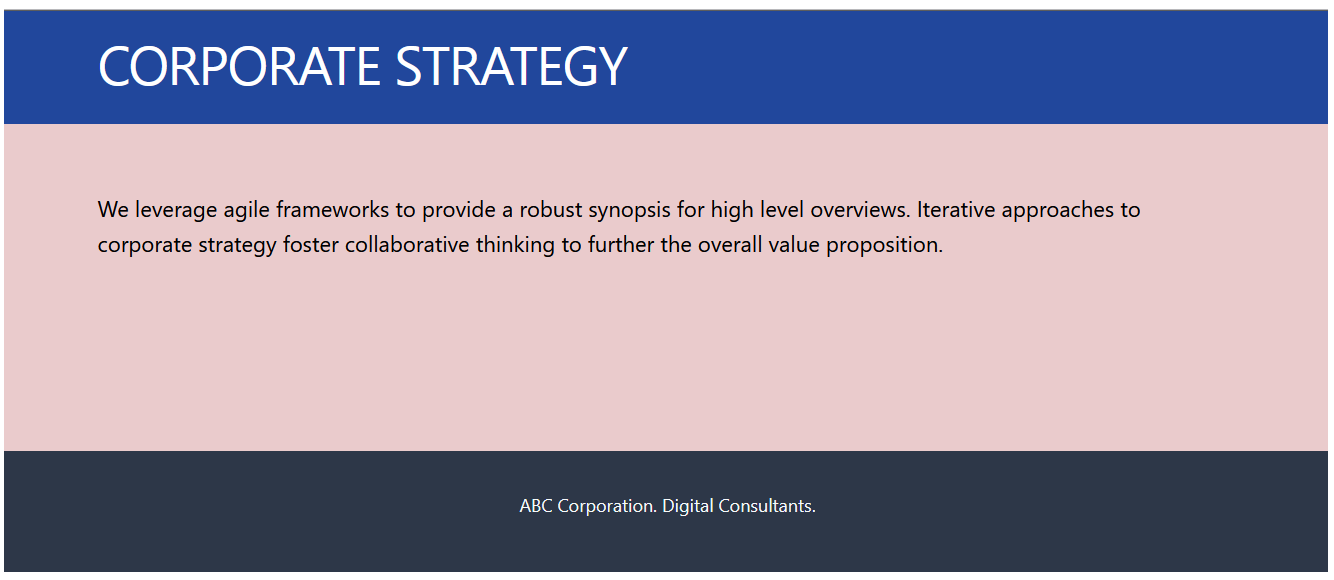
Adding an image file
As with CSS files, it is best to add them from the /src folder with import statements.
The only exceptions might be for generic site-wide images which would be tedious to manually import multiple times into different component files. Or when the React app is only part of a larger website.
You can import an image into your sample app as follows.
- Download the following file to your /src folder. corporate.jpg
- In your Main.js component, add the following import statement at the top of the file.
import corporateImg from './corporate.jpg';
- Now you can reference the image by its variable name. See below.
function Main() { return ( <main> <section> <img src={corporateImg} alt="Corporate" /> <p>We leverage agile frameworks to provide a robust synopsis for high-level overviews. Iterative approaches to corporate strategy foster collaborative thinking to further the overall value proposition.</p> </section> </main> ) }
Your web page should now look as shown below.
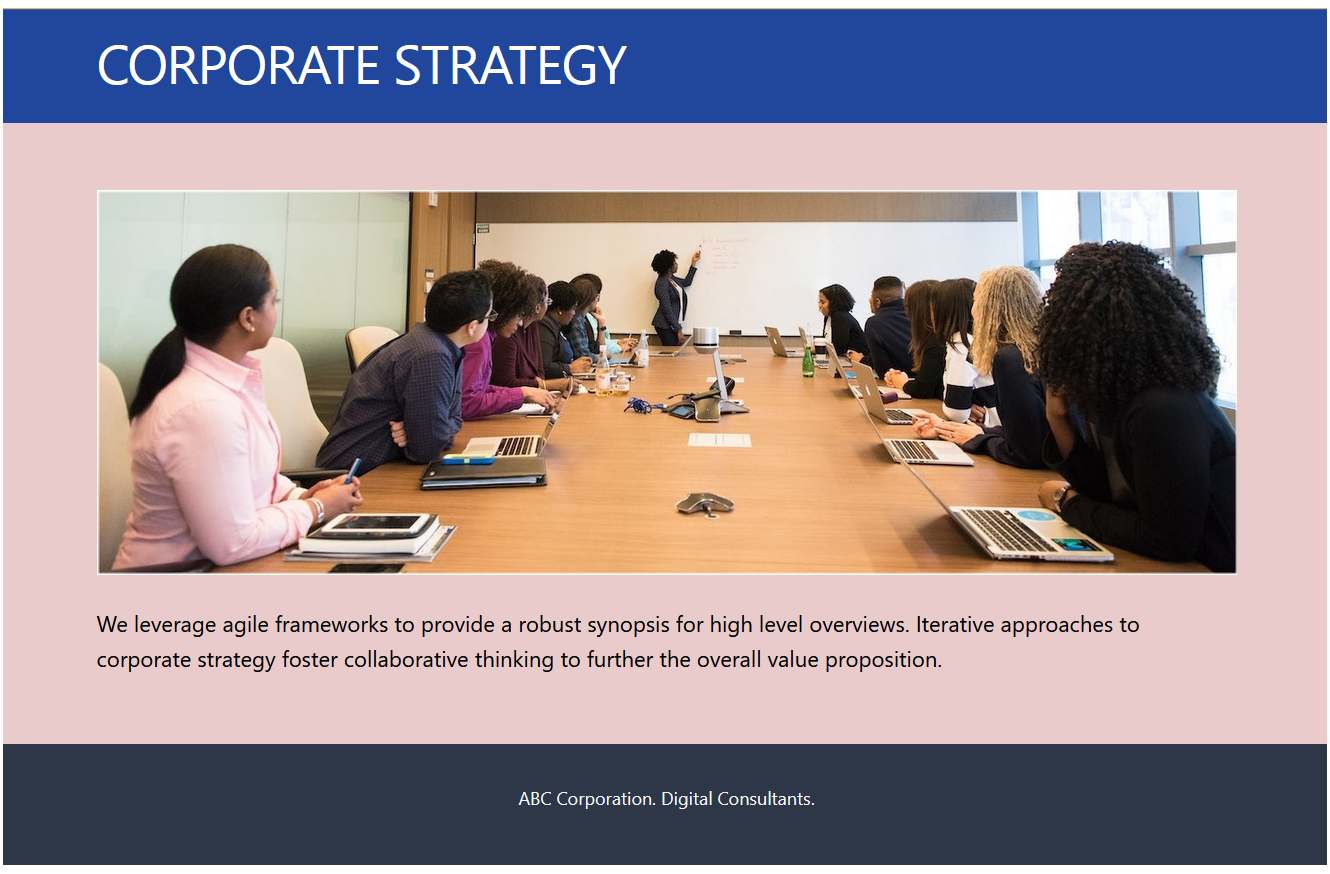