Learning Goals
At the end of this Tutorial, you will be able to:
- Run the create-react-app utility and be familiar with its folder and file structure.
- Customise the template files according to your requirements.
- Build a basic app with functional components, and imported CSS and image files.
About eCommerce app
In this app for an e-commerce store there are three different products with three separate product descriptions. The app will contain the following components:
- A top-level App component at the top of the hierarchy.
- A lower-level Product component, which we will reuse three times. The Product component will be customised using props, passed downward from the Apps component.
- And a still lower-level itemDescription component, also reused three times.
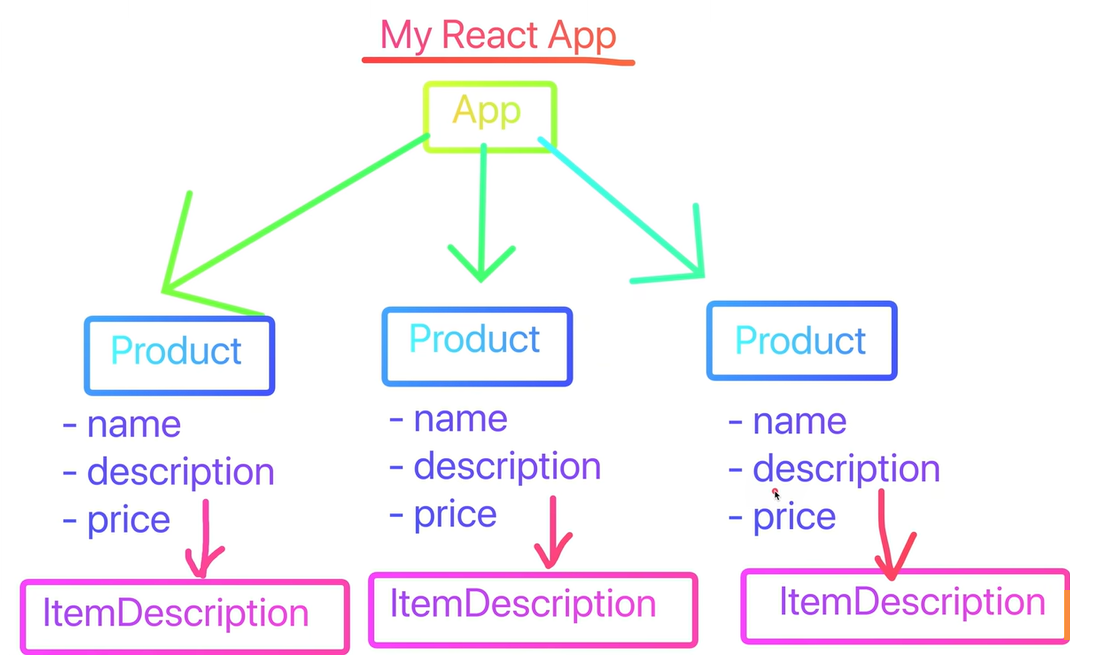
Building with Create React App
Follow these steps to buld the basic app structure with the create-react-app script.
- In VS Code, choose Terminal | New terminal and navigate to where you want React to create a folder for your app. For example:
C:\> users\JohnSmith
orC:\>
- Type the following command that includes the name you want to call your new app.
C:\> npx ecommerce-reactjs
After you have created your app, you launch it by running the following command from inside your app folder.
C:\ecommerce-reactjs> npm start
A new browser window should display with your new app running on the React local development server. If not, open a new browser tab and enter http://localhost:3000.
You will see a screen similar to the following.
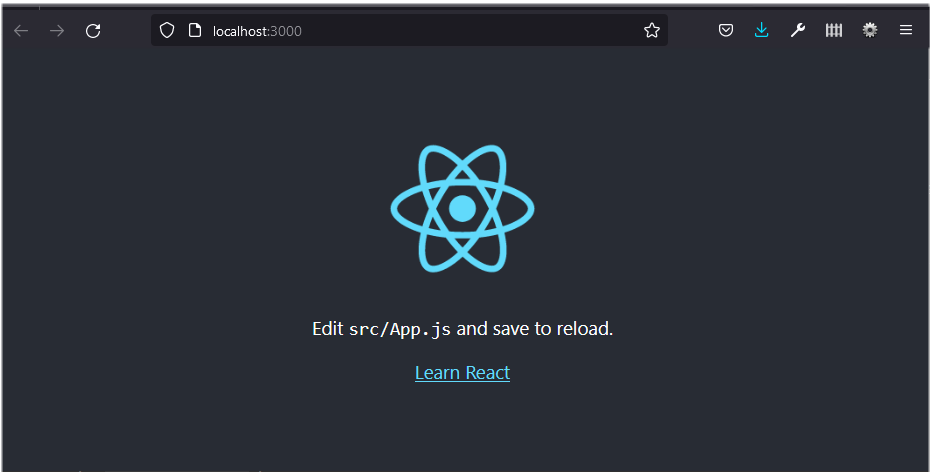
Customising your app
In this section, you will customise the 'boilerplate' content provided by the create-react-app script to design your ecommerce app.
- Open the index.html file in the top-level folder and replace all its content with the following.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <title>eCommerce React App</title> <meta name="description" content="eCommerce website created with React.js" /> <link rel="manifest" href="%PUBLIC_URL%/manifest.json" /> </head> <body> <div id="root"></div> </body> </html>
- Open the index.js file in the /src folder and replace all its content with the following.
import React from 'react'; import ReactDOM from 'react-dom/client'; import App from './App'; const root = ReactDOM.createRoot(document.getElementById('root')); root.render( <React.StrictMode> <App /> </React.StrictMode> );
- Open the App.js file in the /src folder and replace its content with the following.
import React from 'react'; import Product from './Product'; function App() { return ( <div> <h1>Hello, World!</h1> <Product name="Google Home" description="Your AI Assistant" price="${59}" /> <Product name="iPhone 15 Pro Max" description="The best Apple iPhone ever" price="${1200}" /> <Product name="Apple MacBook Pro" description="A really nice laptop" price="${2500}" /> </div> ); }
- In the /src folder, create a new, empty file named Product.js and enter the following content to it.
import React from 'react'; function Product(props) { return ( <div> <h1>Hello, World!</h1> <h1>{props.name}</h3> <h2>{props.description}</h2> <h3>${props.price}</h3> </div> ); } export default Product;
- Rewrite the function component to use object destructuring as follows:
function Product({ name, description, price }) { return ( <div> <h1>{name}</h3> <h2>{description}</h2> <h3>${price}</h3> </div> ); }
- Inside this Product component, nest a new component named ItemDescription as shown below.
function Product({ name, description, price }) { return ( <div> <h1>{name}</h3> <h2>{description}</h2> <h3>${price}</h3> <ItemDescription /> </div> ); }
- Add an import statement at the top of your App.js file.
import ./ItemDescription
- In the /src folder, create a new, empty file named ItemDescription.js and enter the following content to it.
import React from 'react'; function ItemDescription( {name, description } ) { return ( <div> <p>{name}</p> <p><i>{description}<i></p> </div> ); } export default ItemDescripton
- Return to the Products.js file and update the function as follows.
function Product({ name, description, price }) { return ( <div> <h1>{name}</h3> <h2>{description}</h2> <ItemDescription name="name" description ="description" > <h3>${price}</h3> </div> ); }