Project Goals
In this fifth stage of the MERN project, you will:
- Simplify the two routes to list all products and to retrieve a single product by id.
- Move all the product routes from index.js in the /backend folder to a separate file.
- Creating a frontend configuration .env file to hold the product route URLs.
Updating your home page route
Currently, your frontend home page lists all items from the MongoDB products collection using the http://localhost:5000/read route. Let's update this route to use the http://localhost:5000 base URL instead:
-
In the
frontend/src/pages folder, update the
getProduct() function in the HomePage.jsx file as
follows:
const getProduct = async () => { try { const response = await axios.get("http://localhost:5000"); setProductList(response.data); console.log(response.data); } catch (e) { console.log(e); } };
-
In the backend/src/ folder, in the index.js file,
update the get all products route as follows:
// Get the all product list app.get("/", async (req, res) => { const productList = await Product.find(); res.send(JSON.stringify(productList)); });
-
Also in this index.js file, delete the test message:
- Using POSTMAN or similar, verify that your new list all products route is working correctly.
Updating your get product route
Currently, your route for retrieving a single product by id from the MongoDB products collection is the http://localhost:5000/get/<id>. Let's update this route to use the simpler http://localhost:5000/<id> URL instead:
-
In the
frontend/src/components folder, the
getProduct() function currently looks as follows:
-
Update the route as shown below:
const getProduct = async () => { try { const response = await axios.get("http://localhost:5000/" + id); console.log(response.data); setProductData(response.data); } catch (e) { console.log(e); } };
- Using POSTMAN or similar, verify that your get product by id route is working correctly.
Creating a products.js
Routes file
Currently, all your CRUD product routes are in the main backend index.js file. Let's move them to a separate file.
- In your /backend folder, create a new sub-folder named Routes.
-
In this Routes sub-folder, create a new text file named
products.js and add the three import statements below to
the top of the file:
import express from "express"; import mongoose from 'mongoose' import Product from "../Models/products.js"; const router = express.Router();
- Now, cut-and-paste all five CRUD routes from index.js into this products.js file.
-
For each of the five routes, replace the app object by the
router object. See the example below:
-
At the end of the products.js file, add this export
statement:
-
In index.js, remove the following import statement:
-
And add this new import statement:
import productRoutes from "./Routes/products.js";
-
Finally, add the new line below to the middleware part of
index.js:
In this index.js code, you are importing the productRoutes from the products.js file and using them as middleware for the path /products.
Note the plural ‘s’.
This means that any request to the server with a path that starts with /products will be handled by the routes defined in products.js.
You will next need to update the routes in other files to take account of this /products prefix in the URLs.
Adding a frontend .env
file for route URLs
Your CRUD product routes currently contain a hard-coded URL of http://localhost:5000. For example, here is a product route from HomePage.jsx.
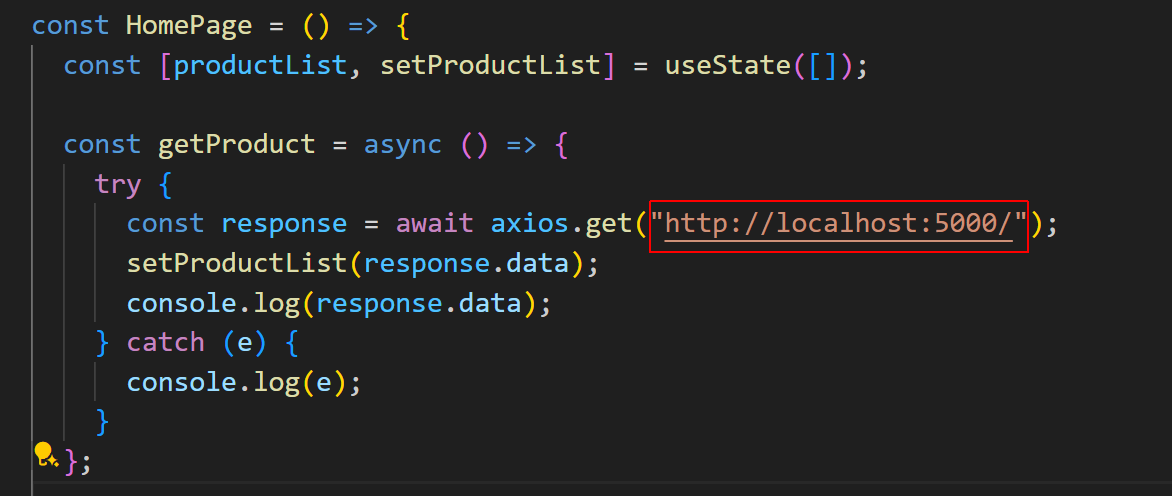
It is better to abstract the URL and move it to a new .env configuration file in your root frontend folder. Follow these steps:
- In your /frontend folder, create a new text file and name it .env.
-
In this new .env file, enter the URL below:
Note that you now two configuration .env files: one in your root /frontend folder and one in your /backend folder. Next, you can update the four frontend files containing the hard-coded URL with this configuration variable. At the same time, you can add the /products prefix to the URLs.VITE_BASE_URL=http://localhost:5000
-
In frontend/src/pages, in HomePage.jsx, update the
hard-coded route within the getProduct function as shown
below:
const response = await axios.get(`${baseURL}/products`);
-
In frontend/src/components, in AddProduct.jsx, the
handleSave function looks as follows:
Update the function as shown below:
const response = await axios.post(`${baseURL}/products/create`, { data: productData, });
-
In frontend/src/components, in UpdateProduct.jsx,
the getProduct function looks as follows:
Update the hard-coded URL as shown below:
In the same file, the handleUpdate function currently looks as follows:const response = await axios.get(`${baseURL}/products/${id}`);
Update the hard-coded URL as shown below:
const response = await axios.put(`${baseURL}/products/update/${id}`, { data: productData, });
-
In frontend/src/components, in ProductCard.jsx, the
handleDelete function looks as follows:
Update the hard-coded URL as shown below:
const response = await axios.delete(`${baseURL}/products/delete/${id}`);
-
Finally, for all four of the above files, add the following two
lines after the import statements:
const baseURL = import.meta.env.VITE_BASE_URL; axios.defaults.baseURL = baseURL;
Because you have added a new .env file, you will need to restart your frontend ReactJS Vite server.
Project checklist and next step
Before continuing:
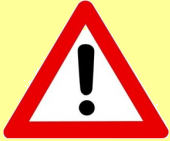
CHECK that your updated CRUD routes for creating, reading, updating, and deleting products are working correctly.
You are now ready to
deploy your MERN app to
GitHub.