Learning Goals
At the end of this Tutorial, you will be able to:
- Pass props down from parent to child components.
About props in React
Props (short for 'properties') are arguments passed down from a parent component to its child component.
For example, you could define your child component to accept props as an input parameter.
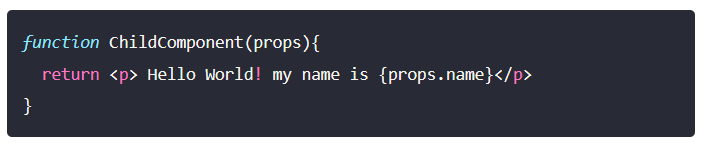
And in your parent component you pass arguments to that child component as follows.

Adding props as parameters to components
Follow these steps to add some props to the basic app you built with create-react-app in the previous Tutorial.
- In VS Code, choose Terminal | New terminal and use npm start to launch your app. For example:
- From the /src folder of your app, open the Main.js component file.
- Near the start of the text paragraph, add the prop {companyName} as shown below.
- Also from the /src folder, open the Footer.js component file.
- Add the two props {publishMonth} and {publishYear} as shown below.
Passing arguments to props
Your next step is to pass values as arguments to the props in your components when the components are called by the top-level App.js component.
- From the /src folder of your app, open the top-level App.js component file.
- Add arguments that will be passed to your components as follows.
Your web page should now look as shown below.
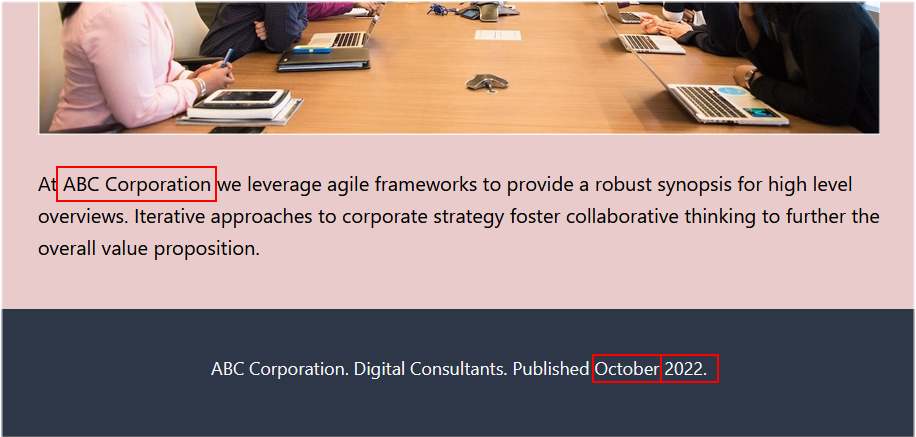
Props and data types
Only strings can be passed to components without curly braces {}.
For numeric and boolean values, and for arrays, enclose them in one pair of curly braces {}.
For objects, use two pairs of curly braces {{}}.
As an example, add the following inside the <section> tag to your Main.js component file.
<p>The <b>Text</b> is: {props.str}</p> <p>The <b>Number</b> is: {props.num}</p> <p>The <b>Truth</b> is {props.truth}</p> <p>The <b>Array</b> is: {props.arr[0]}</p> <p>The <b>username</b> in <b>users</b> object is: {props.obj.name}</p>
And update the <Main /> component inside your App.js top-level component as follows.
<Main props.num = {100}; companyName="ABC Corporation" str ="Hello" num={42} truth={true} arr={[99]} obj={{name: 'Alice'}} />
You should see the following output on the web page.
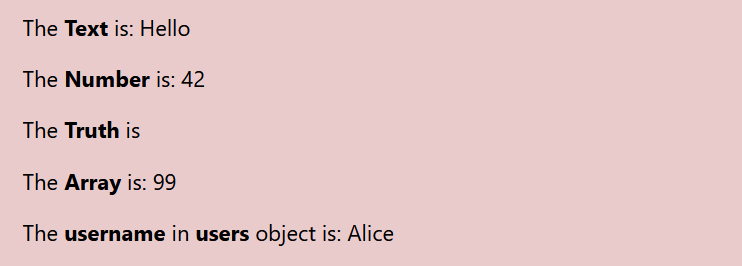
In real-world apps, the child component often renders conditionally depending on whether or not the props are passed into it. See below.
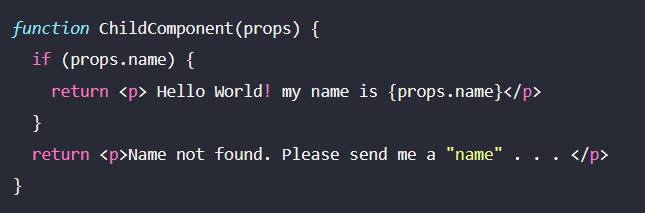
Props are Read-Only
In React, a functional component can never modify its own props. Consider this JavaScript sum function:
function sum(a, b) { return a + b; }
Such functions are called pure because they do not attempt to change their inputs, and always return the same result for the same inputs.
In contrast, this function is impure because it changes its own input:
function updatePrice(amount) { amount = amount * 0.15; return amount; }
All React components must act like pure functions with respect to their props.
The following example will still output whatever value is passed down to it from its parent component instead of “Mark”:
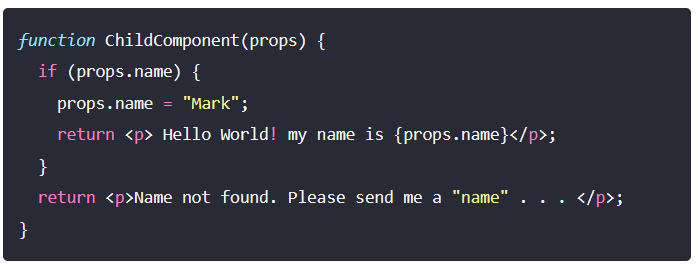